Using `onViewableItemsChanged`
You might want to know viewable items in a FlatList
and are notified when viewable items are changed. For example: in list of videos, you want to automatically play a video when the video when it appears on the screen for a few seconds.
In Android, you can use findFirstVisibleItemPosition and findLastVisibleItemPosition if your RecyclerView is using LinearLayoutManager or GridLayoutManager. Besides, it provides isViewPartiallyVisible to claim if a child view is partially or fully visible. In iOS, you could use visibleCells
in UITableView
. React Native's FlatList
component has its own powerful property: onViewableItemsChanged
. This guide will show you how to use the onViewableItemsChanged
and how it works under the hood.
onViewableItemsChanged
What is onViewableItemsChanged
is a prop accessible to both VirtualizedList and FlatList components. When you scroll one of these lists, only a few list items can be seen on screen at any time. These visible items are the viewableItems
. When the onViewableItemsChanged
function is called, it returns with an array of the currently visible items, viewableItems
, and an array of the items that are no longer visible, changed
items.
How to use it
In this example, you will learn how to use [onViewableItemsChanged](virtualizedlist#onviewableitemschanged)
and [ViewabilityConfig](virtualizedlist#viewabilityconfig)
in FlatList.
- onViewableItemsChanged Example
- viewabilityConfigCallbackPairs Example
onViewableItemsChanged
should be used together with ViewabilityConfig
. ViewabilityConfig
is the configuration you customize when the onViewableItemsChanged
is called. onViewableItemsChanged
is called when its corresponding ViewabilityConfig
's conditions are met.
<FlatList
viewabilityConfig={viewabilityConfig}
onViewableItemsChanged={onViewableItemsChanged}
{...}
/>
viewabilityConfig = {
waitForInteraction: true,
itemVisiblePercentThreshold: 75,
minimumViewTime: 800
};
In this snippet, waitForInteractions is true. If waitForInteractions
is set to true, only when the user scrolls or recordInteraction
is called, FlatList
will calculate the viewable items.
waitForInteraction: true,
Additionally, minimumViewTime
is set to 800, and itemVisiblePercentThreshold
is set to 75. This config means the item is marked as visible only if 75% or more of it is physically viewable for more than 800 milliseconds.
itemVisiblePercentThreshold: 75,
minimumViewTime: 800,
Supposed you have to do different things for items with 60% viewable region and those with 75% viewable region. You can use viewabilityConfigCallbackPairs
, which is a list of ViewabilityConfig
/onViewableItemsChanged
pairs. In the following code, on60ViewableItemsChanged
will be called when some items are at least 60% viewable for more than 600 milliseconds. In the meanwhile, on75ViewableItemsChanged
will be called when some items are at least 75% viewable for more than 700 milliseconds.
viewabilityConfigCallbackPairs = [
{
viewabilityConfig: {
minimumViewTime: 600,
itemVisiblePercentThreshold: 60
},
onViewableItemsChanged: on60ViewableItemsChanged
},
{
viewabilityConfig: {
minimumViewTime: 700,
itemVisiblePercentThreshold: 75
},
onViewableItemsChanged: on75ViewableItemsChanged
}
];
onViewableItemsChanged
works
How Viewable Region
The layout and viewable region information for VirtualizedList
is stored in a _scrollMetrics
object. Through the nativeEvent
in onScroll
callback, VirtualizedList
can access this layout information.
const timestamp = e.timeStamp;
let visibleLength = this._selectLength(
e.nativeEvent.layoutMeasurement
);
let contentLength = this._selectLength(e.nativeEvent.contentSize);
let offset = this._selectOffset(e.nativeEvent.contentOffset);
let dOffset = offset - this._scrollMetrics.offset;
// ... more code here
this._scrollMetrics = {
contentLength,
dt,
dOffset,
offset,
timestamp,
velocity,
visibleLength
};
If it is a vertical VirtualizedList
, the layout.layoutMeasurement.height
in the nativeEvent
is assigned to visibleLength
, the height of viewable region.
Overview
viewabilityConfig
in one VirtualizedList
Different _viewabilityTuples
is an array inside VirtualizedList
to store ViewabilityHelper/onViewableItemsChanged
pairs. This array is initialized in the constructor
function.
_viewabilityTuples: Array<ViewabilityHelperCallbackTuple> = [];
type ViewabilityHelperCallbackTuple = {
viewabilityHelper: ViewabilityHelper,
onViewableItemsChanged: (info: {
viewableItems: Array<ViewToken>,
changed: Array<ViewToken>,
...
}) => void,
...
};
If you define viewabilityConfigCallbackPairs, each viewabilityConfig
will be used to initialize a different ViewabilityHelper
object.
if (this.props.viewabilityConfigCallbackPairs) {
this._viewabilityTuples = this.props.viewabilityConfigCallbackPairs.map(
(pair) => ({
viewabilityHelper: new ViewabilityHelper(
pair.viewabilityConfig
),
onViewableItemsChanged: pair.onViewableItemsChanged
})
);
} else if (this.props.onViewableItemsChanged) {
this._viewabilityTuples.push({
viewabilityHelper: new ViewabilityHelper(
this.props.viewabilityConfig
),
onViewableItemsChanged: this.props.onViewableItemsChanged
});
}
ViewabilityHelper
is a utility class for calculating viewable items based on the viewabilityConfig and metrics, like the scroll position and layout.
As I mentioned before, in a VirtualizedList
could has several ViewabilityHelper
objects in _viewabilityTuples
, containing different viewabilityConfig
to handle different viewability conditions. Here are some important props in ViewabilityHelper
.
class ViewabilityHelper {
_config: ViewabilityConfig;
_hasInteracted: boolean = false;
/* A set of `timeoutID`, used for memory management */
_timers: Set<number> = new Set();
// Indexes of the viewable items
_viewableIndices: Array<number> = [];
// A map for viewable items
_viewableItems: Map<string, ViewToken> = new Map();
}
Items' layout
In the overview graph, you can see a func _updateViewableItems
called in many scenarios. For example, it is called in onScroll
callback. Then, It calls viewabilityHelper.onUpdate
to find out the viewable items, which appear in the viewport for VirtualizedList.
_updateViewableItems(data: any) {
const {getItemCount} = this.props;
this._viewabilityTuples.forEach(tuple => {
tuple.viewabilityHelper.onUpdate(
getItemCount(data),
// contentOffset of the list
this._scrollMetrics.offset,
// 🌟 viewportHeight
this._scrollMetrics.visibleLength,
this._getFrameMetrics,
this._createViewToken,
tuple.onViewableItemsChanged,
this.state,
);
});
}
this._scrollMetrics.visibleLength
is used asviewportHeight
this._createViewToken
is used to construct aViewToken
object, which containsitem
data,index
,key
andisViewable
flag of theitem
.- this._getFrameMetrics is a function to get layout information of the item cell by index. The item layout is from
getItemLayout
prop ofVirtualizedList
orthis._frames
map.this._frames
stores the itemKey/itemLayout pairs.
// this._frames stores the item cell layout info
{ [cellKey]: {
// offset of the item cell
offset: number,
// length of the item cell. width or height determined by the direction of the VirtualizedList
length: number,
index: number,
inLayout: boolean,
}
}
- By
this.state
, you know the range of the rendered items byfirst
andlast
value.VirtualizedList
updates these two values when the rendered items are changed.
type State = {
// The range of the rendered items,
// used for the optimization to reduce the scan size
first: number,
last: number,
...
};
How to find out viewable items
In onUpdate
method, it calls computeViewableItems
to get viewableIndices
. viewableIndices
is an array of indexes of the viewable items. So, how does computeViewableItems
work?
How to get the indexes of viewable items
In computeViewableItems
in the ViewabilityHelper
class, it iterates items from ${first}
to ${last}
. If an item is viewable, it will be stored in an array named viewableIndices
.
for (let idx = first; idx <= last; idx++) {
const metrics = getFrameMetrics(idx);
if (!metrics) {
continue;
}
// The top of current item cell, relative to the screen coordinate
const top = metrics.offset - scrollOffset;
// The bottom of current item cell, relative to the screen coordinate
const bottom = top + metrics.length;
if (top < viewportHeight && bottom > 0) {
firstVisible = idx;
if (
_isViewable(
viewAreaMode,
viewablePercentThreshold,
top,
bottom,
viewportHeight,
metrics.length,
)
) {
viewableIndices.push(idx);
}
} else if (firstVisible >= 0) {
break;
}
}
return viewableIndices;
}
From the code, you can see the top
and bottom
value is related to the screen coordinate. I drew a graph to show the relationship between metrics.offset
, scrollOffset
, metrics.length
, top
and bottom
. This graph will help you better understand the above code.
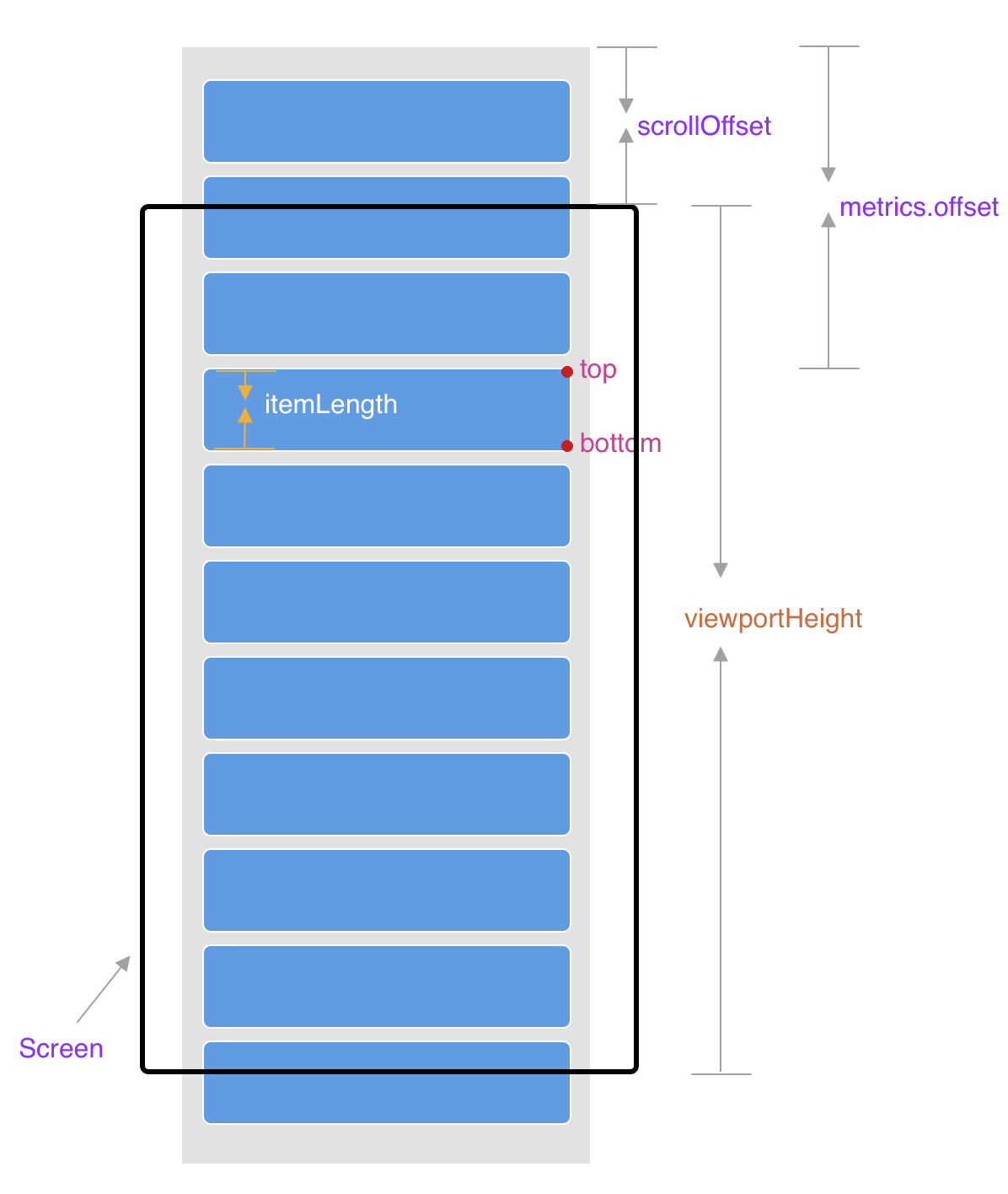
What kind of item is viewable
An item is said to be viewable when it meets the following conditions for longer than ${minimumViewTime}
milliseconds (after an interaction if waitForInteraction
is true):
- the fraction of the item visible in the view area >=
itemVisiblePercentThreshold
. When it comes to the fraction of the item visible in the view area, you need to take care about cases shown in the following graph. RN useMath.min(bottom, viewportHeight) - Math.max(top, 0)
to calculate the viewable length.
- Entirely visible on screen when the height of a item is bigger than the
viewportHeight
.
function _isViewable(
viewAreaMode: boolean,
viewablePercentThreshold: number,
top: number,
bottom: number,
viewportHeight: number,
itemLength: number
): boolean {
if (_isEntirelyVisible(top, bottom, viewportHeight)) {
// Entirely visible
return true;
} else {
// Get viewable height of this item cell
const pixels = _getPixelsVisible(top, bottom, viewportHeight);
// Get the viewable percentage of this item cell
const percent =
100 *
(viewAreaMode
? pixels / viewportHeight
: pixels / itemLength);
return percent >= viewablePercentThreshold;
}
}
function _getPixelsVisible(
top: number,
bottom: number,
viewportHeight: number
): number {
const visibleHeight =
Math.min(bottom, viewportHeight) - Math.max(top, 0);
return Math.max(0, visibleHeight);
}
function _isEntirelyVisible(
top: number,
bottom: number,
viewportHeight: number
): boolean {
return top >= 0 && bottom <= viewportHeight && bottom > top;
}
_isViewable
source code is here
Timer and Schedule
In onUpdate
func in ViewabilityHelper, if you define minimumViewTime
value, the _onUpdateSync
is scheduled to be called. It is the handler of the timeout
.
this._viewableIndices = viewableIndices;
if (this._config.minimumViewTime) {
const handle = setTimeout(() => {
this._timers.delete(handle);
// filter out indices that have gone out of view after minimumViewTime
// figure out which items are gone, which items are showing
this._onUpdateSync(
viewableIndices,
onViewableItemsChanged,
createViewToken
);
}, this._config.minimumViewTime);
this._timers.add(handle);
} else {
this._onUpdateSync(
viewableIndices,
onViewableItemsChanged,
createViewToken
);
}
If some items aren't longer viewable after a few seconds, ${minimumViewTime}, the _onUpdateSync func filters out these indices that have gone out of viewport.
// Filter out indices that have gone out of view after `minimumViewTime`
viewableIndicesToCheck = viewableIndicesToCheck.filter((ii) =>
this._viewableIndices.includes(ii)
);
In the above graph, at first, the _viewableIndices
is from 1 to 9. Then the user scrolls the VirtualizedList
and the _onUpdateSync
is triggered after minimumViewTime
. At this moment, the current _viewableIndices
is from 2 to 10. So the item indexed 1 is filtered out.
How to get changed items
Comparing with the last time when onViewableItemsChanged
is triggered, at this time to trigger onViewableItemsChanged
. Some viewable items will be out of the screen, some hidden items will become viewable. In _onUpdateSync
function, the preItems
map stores the information about previous visible items, the previous means last time when VirtualizedList
calls onViewableItemsChanged
. Now it has a nextItems
map, which stores the information about viewable items this time. Then it figures out the changed
items by comparing these two maps. Then, it calls onViewableItemsChanged
, passing viewableItems
and changed
items.
_onUpdateSync(
viewableIndicesToCheck,
onViewableItemsChanged,
createViewToken,
) {
// Filter out indices that have gone out of view since this call was scheduled.
viewableIndicesToCheck = viewableIndicesToCheck.filter(ii =>
this._viewableIndices.includes(ii),
);
const prevItems = this._viewableItems;
// Using map, so the time complexity would be o(n)
const nextItems = new Map(
viewableIndicesToCheck.map(ii => {
const viewable = createViewToken(ii, true);
return [viewable.key, viewable];
}),
);
const changed = [];
for (const [key, viewable] of nextItems) {
if (!prevItems.has(key)) {
changed.push(viewable);
}
}
for (const [key, viewable] of prevItems) {
if (!nextItems.has(key)) {
changed.push({...viewable, isViewable: false});
}
}
if (changed.length > 0) {
this._viewableItems = nextItems;
onViewableItemsChanged({
viewableItems: Array.from(nextItems.values()),
changed,
viewabilityConfig: this._config,
});
}
}
}